How To Create An Object Js
Summary: in this tutorial, you will learn various patterns to create objects in JavaScript and understand the pros and cons of each.
In the previous tutorial, you have learned how to create JavaScript objects using the object literal syntax.
The object literal syntax is convenient for creating a single object. In case you want to create multiple similar objects, you need to use one of the following patterns:
- Factory pattern
- Constructor pattern
- Prototype pattern
- Constructor / prototype pattern
- Parasitic constructor pattern
- Durable constructor pattern
Factory pattern
The factory pattern uses a function to abstract away the process of creating specific objects.
For example, the following createAnimal()
function encapsulates the logic of creating the animal
object.
Code language: JavaScript ( javascript )
// factory pattern function createAnimal(name) { var o = new Object(); o.name = name; o.identify = function() { console.log("I'm " + o.name); } return o; }
The createAnimal()
function accepts an argument that will be used to initialize the name
property of the animal
object.
To create a new object, you just need to call this function and pass the name
argument as follows:
Code language: JavaScript ( javascript )
var tom = createAnimal('Tom'); var jerry = createAnimal('Jerry'); tom.identify(); // I'm Tom jerry.identify(); // I'm Jerry
Although the factory pattern can create multiple similar objects, it doesn't allow you to identify the type of object it creates.
Constructor pattern
JavaScript allows you to create a custom constructor function that defines the properties and methods of user-defined objects.
By convention, the name of a constructor function in JavaScript starts with an uppercase letter.
For example, the following rewritten the animal
object of the prior example:
Code language: JavaScript ( javascript )
function Animal(name) { this.name = name; this.identify = function() { console.log("I'm " + this.name); }; }
Unlike the factory pattern, the properties and methods of the animal
object are assigned directly to the this
object inside the constructor function.
At this point, JavaScript engine creates the Animal()
function and an anonymous object.
The Animal()
function has the prototype
property reference an anonymous object and the anonymous object has the constructor
property reference the Animal()
function.
In addition, the JavaScript engine links the anonymous object to the Object.
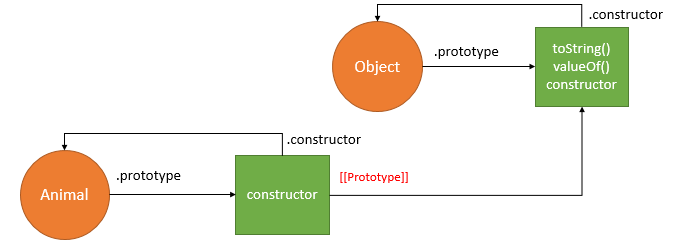
To create a new instance of Animal
, you use the new
operator. For example:
Code language: JavaScript ( javascript )
var donald = new Animal('Donald');
Behind the scenes, JavaScript executes these four steps:
- Create a new object.
- Set the
this
value of the constructor to thenew
object. - Execute code inside the constructor i.e., adding properties to the new object.
- Return the new object.
The following figure illustrates the relationship between thedonald
object and other objects.
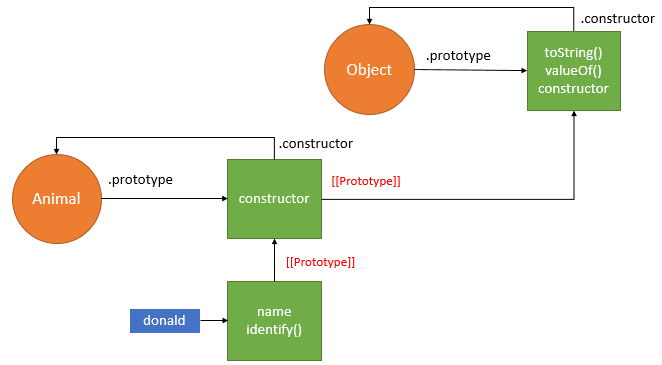
See the following example.
Code language: JavaScript ( javascript )
console.log(donald.constructor === Animal); // true
In this example, because thedonald
object does not have the constructor
property, JavaScript engine follows the prototype chain to find it in the Animal.prototype
object.
It found the constructor
property in the Animal.prototype
object and in this case the constructor
property points to the Animal()
function, therefore the statement above returns true
.
Thedonald
object is also an instance of Animal
andObject
as follows:
Code language: JavaScript ( javascript )
console.log(donald instanceof Animal); // true console.log(donald instanceof Object); // true
The disadvantage of the constructor pattern is that the same method identify()
is duplicated in each instance.
The following code creates a new Animal
object named bob
.
Code language: JavaScript ( javascript )
var bob = new Animal('Bob');
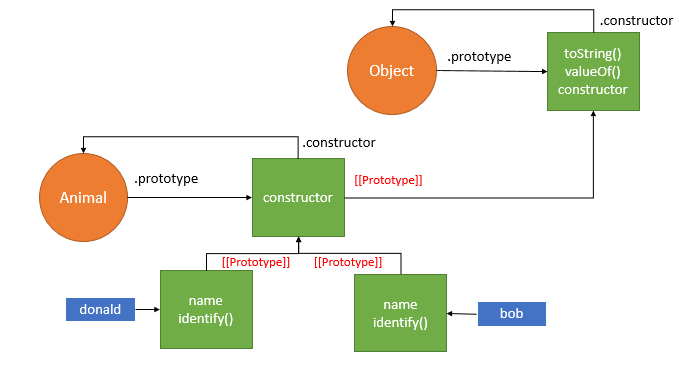
As you see, the identify()
method is duplicated in bothdonald
and bob
objects. To solve this issue, you use the prototype pattern.
Prototype pattern
The prototype pattern adds the properties of the object to the prototype object. Then, these properties are available and shared among all instances.
The following example uses the prototype pattern to rewrite the Animal object above.
Code language: JavaScript ( javascript )
function Animal() { // properties are added to prototype } Animal.prototype.name = 'Noname'; Animal.prototype.identify = function() { console.log("I'm " + this.name); }
Let's create a new instance of the Animal
.
Code language: JavaScript ( javascript )
var donald = new Animal(); donald.name = 'Donald'; // shadow the name property donald.identify(); // I'm Donald
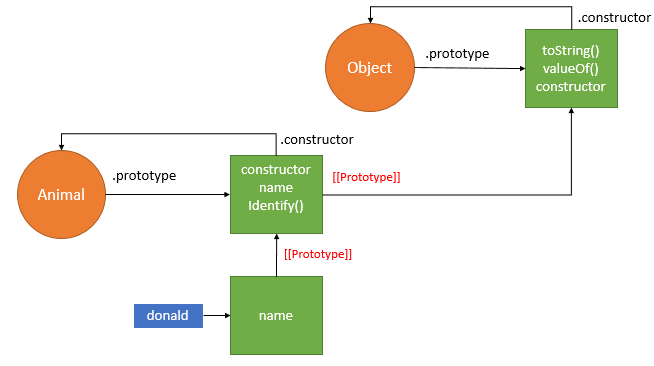
In the line:
Code language: JavaScript ( javascript )
donald.name = 'Donald'; // shadow the name property
JavaScript engine adds the name property to thedonald
object. As the result, bothdonald
and Animal.prototype objects has the same name property.
Inside the identify()
method, the this
object is set to thedonald
object, therefore this.name references to the name property of thedonald
object. As the result, the output of the following line:
Code language: CSS ( css )
donald .identify();
is the string I'm Donald
Let's remove the name property of thedonald
object.
Code language: JavaScript ( javascript )
delete donald.name;
and call the identify()
method again.
Code language: JavaScript ( javascript )
donald.identify(); //I'm Noname
Now, JavaScript could not find the name property in the donald
object, it follows the prototype chain and finds it in the Animal.prototype
object. Hence, the this.name
returns Noname
.
For more information on the prototype, check it out the JavaScript prototype tutorial.
Constructor / Prototype pattern
The combination of the constructor and prototype patterns is the most common way to define custom types.
The constructor pattern defines object properties, while the prototype pattern defines methods and shared properties.
By using this pattern, all objects of the custom type share the method and each of them has its own properties. This constructor/prototype takes the best parts of both constructor and prototype patterns.
Code language: JavaScript ( javascript )
function Animal(name) { this.name = name; } Animal.prototype.identify = function() { console.log("I'm " + this.name); } var donald = new Animal('Donald'); donald.identify(); // I'm Donald var bob = new Animal('Bob') bob.identify(); // I'm Bob
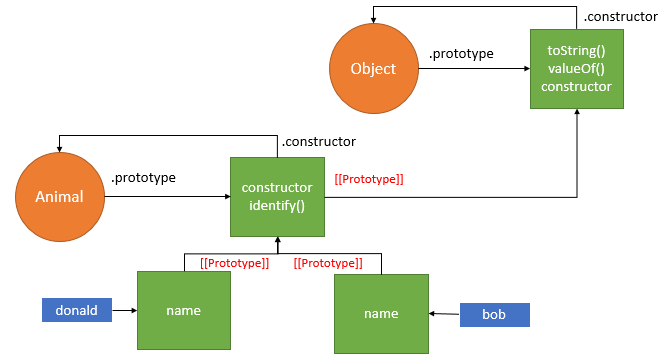
The following figure illustrates the relationships between objects in the constructor / prototype pattern.
Parasitic constructor pattern
In a parasitic constructor pattern, you create a constructor function that creates an object and returns that object.
Code language: JavaScript ( javascript )
function Animal(name) { var o = new Object(); o.name = name; o.identify = function() { console.log("I'm " + o.name); } return o; }
In this example, the Animal constructor function is the same as the one in the factory pattern. However, you call it as a constructor using the new
operator.
Code language: JavaScript ( javascript )
var dog = new Animal('Bingo');
By default, the new
operator returns the object returned by the constructor
function. If the constructor
function does not return an object, the new
operator creates that object instead.
Durable constructor pattern
A durable object is an object that does not have public property and its methods don't reference to the this
object.
The durable objects are often used in secure environments where accessing this
and new
are not allowed.
See the following example:
Code language: JavaScript ( javascript )
function secureAnimal(name) { var o = new Object(); o.identify = function() { console.log(name); // no this } return o; } var alien = secureAnimal('?#@'); alien.identify(); // ?#@
The alien
is a durable object that does not allow the external code to access its members without calling its methods.
In this tutorial, you have learned various patterns to create objects in JavaScript. Each pattern has pros and cons, choosing the appropriate one depends on your programming context.
Was this tutorial helpful ?
How To Create An Object Js
Source: https://www.javascripttutorial.net/create-objects-in-javascript/
Posted by: masseywicis1978.blogspot.com
0 Response to "How To Create An Object Js"
Post a Comment